Strapi populate REST API tutorial (with examples)
— Strapi — 3 min read
How to populate Strapi REST API
Key Concepts
By default, the Strapi REST API will not populate relations. You can read more about it on Strapi docs.
Strapi populate deep example
Let's use Youtube as an example for the relations. On youtube a channel can have many videos, one video can have many comments,and every comment can have many likes.
- The Channel has many Videos
- a Video has many Comments
- a Comment has many Likes
Setup relations with the admin panel
Go to the Content-Type Builder, then create a new collection type with the display name of Channel, you should get channel as singular API ID & channels as plural API ID.
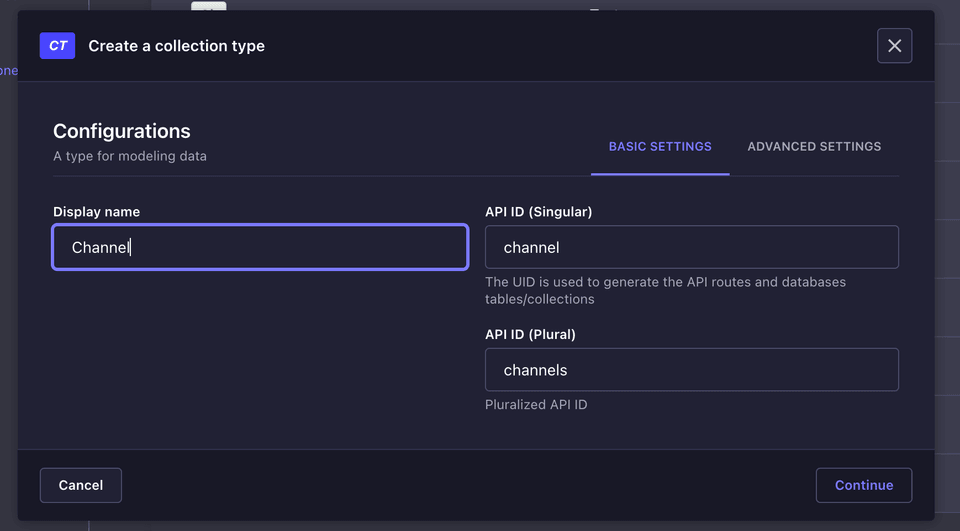
Add new Text field called "title" that will hold the channel name. Then do the same for Videos, Comments and Likes.
After creating the collection, add another field with a Relation type of "has many" to the parent collection.
In Video collection, add "Channel has many Videos" relation.
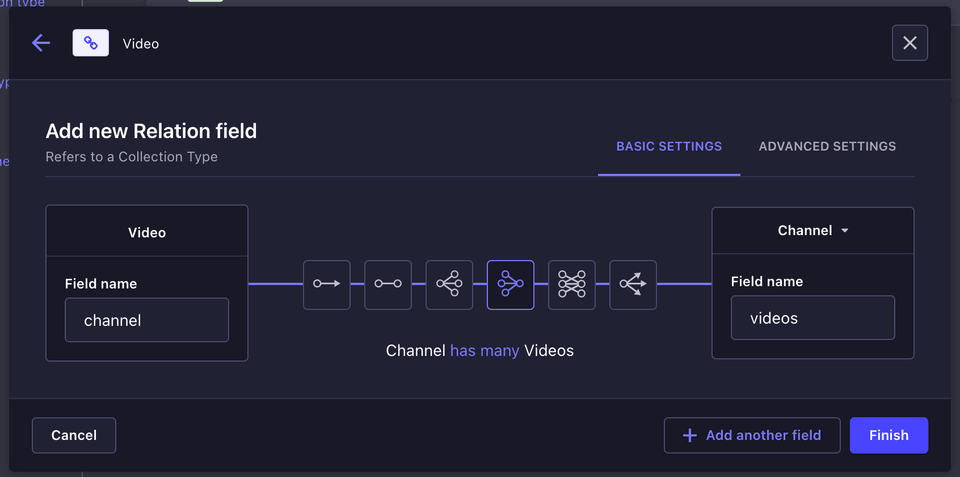
And it will automatically creates "belongs to many" relation in the Channel collection.
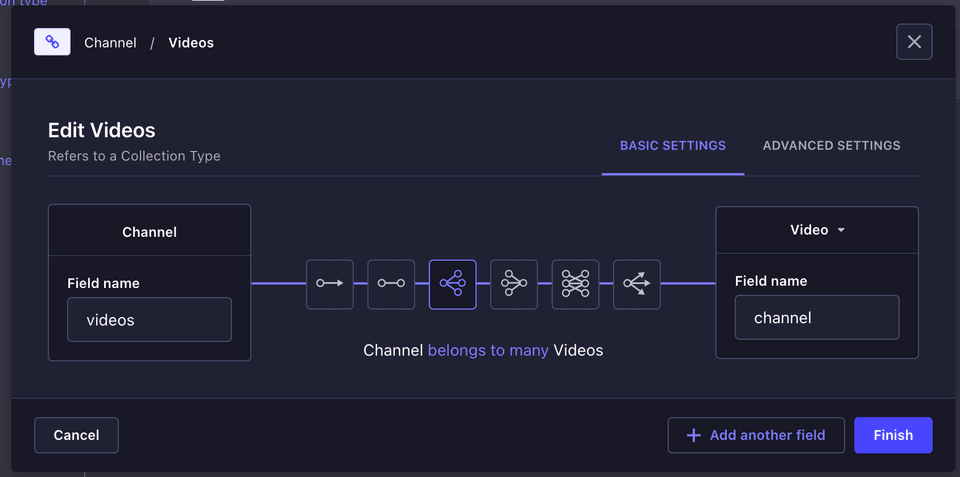
Do the same for Comments relation to Video.
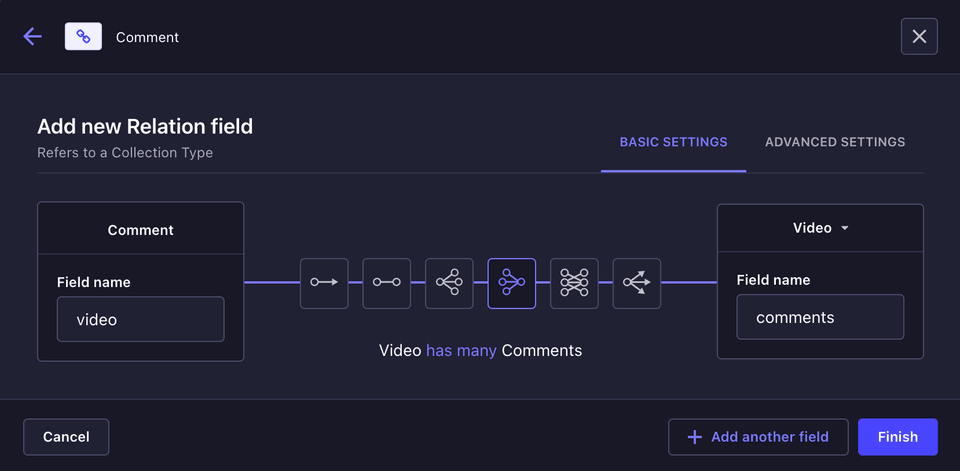
Add content entry
Got to the Content manager, and then add a new entry.
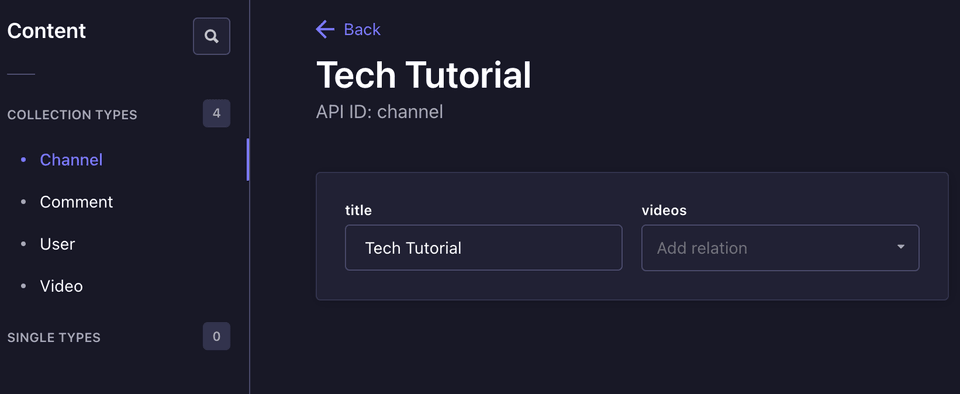
Do the same for Videos, Comments & likes, don't forget configure the relation in the Videos & Comments collection.
Now configure the permissions for the Public role.
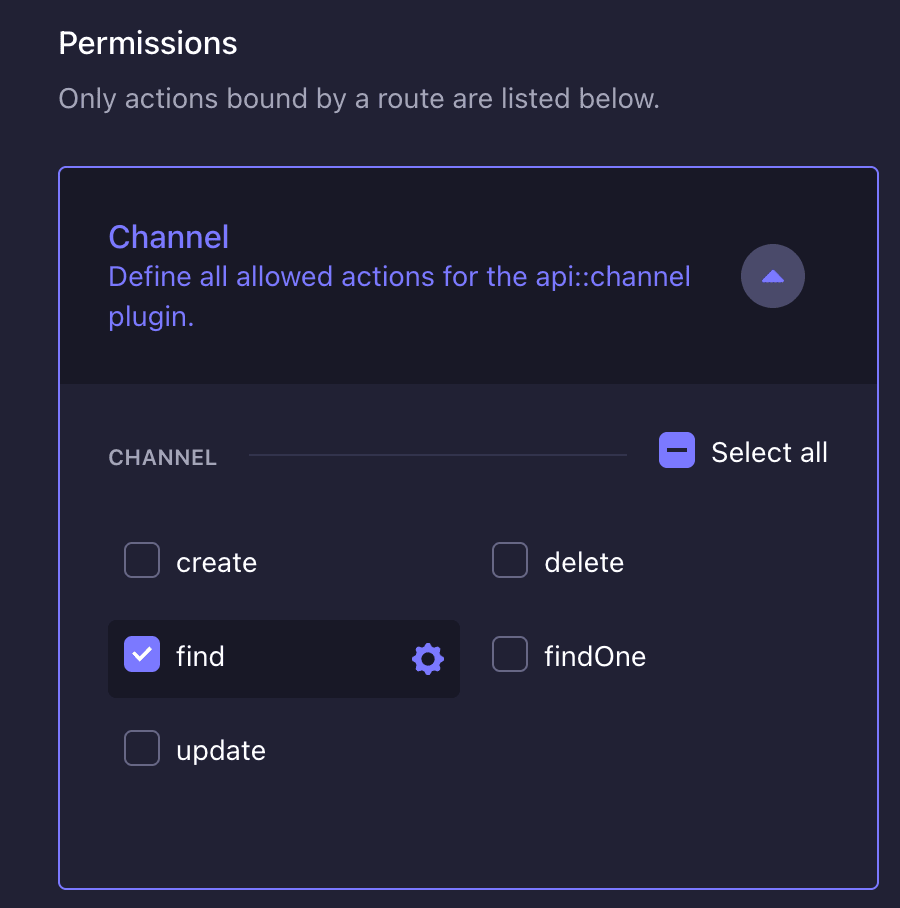
Do the same for the other collections.
You can also manage relations via REST API
Populate relations one level deep example
For the first level you can use this
http://localhost:1337/api/channels?populate=videos
Or * if you want to populate everything on the first level.
http://localhost:1337/api/channels?populate=*
In Strapi 4 you can access video title with
const videoTitle = response.data[0].attributes.videos.data[0].attributes.title;
In Strapi 5 you can access video title with
const videoTitle = response.data[0].videos.data[0].title;
Populate nested relations two levels deep example
http://localhost:1337/api/channels?populate[videos][populate]=comments
In Strapi 4 you can access comment content with
const commentContent = response.data[0].attributes.videos.data[0].attributes.comments.data[0].attributes.content;
In Strapi 5 you can access comment content with
const commentContent = response.data[0].videos.data[0].comments.data[0].content;
Populate nested relations three levels deep example
http://localhost:1337/api/channels?populate[videos][populate][comments][populate]=likes
In Strapi 4 you can access likes with
const commentLikes = response.data[0].attributes.videos.data[0].attributes.comments.data[0].attributes.likes.data;
In Strapi 5 you can access likes with
const commentLikes = response.data[0].videos.data[0].comments.data[0].likes.data
Complete Strapi 4 response example
{ "data": [ { "id": 1, "attributes": { "title": "Tech Tutorial", "createdAt": "2024-09-20T22:00:51.669Z", "updatedAt": "2024-09-20T22:15:54.531Z", "publishedAt": "2024-09-20T22:15:54.529Z", "videos": { "data": [ { "id": 1, "attributes": { "title": "Python Tutorial", "createdAt": "2024-09-20T22:15:38.301Z", "updatedAt": "2024-09-20T22:15:50.252Z", "publishedAt": "2024-09-20T22:15:50.250Z", "comments": { "data": [ { "id": 1, "attributes": { "content": "Nice!", "createdAt": "2024-09-20T22:26:12.060Z", "updatedAt": "2024-09-20T22:27:07.128Z", "publishedAt": "2024-09-20T22:27:07.126Z", "likes": { "data": [ { "id": 1, "attributes": { "createdAt": "2024-09-20T22:31:45.568Z", "updatedAt": "2024-09-20T22:32:33.583Z", "publishedAt": "2024-09-20T22:32:33.580Z" } } ] } } } ] } } } ] } } } ], "meta": { "pagination": { "page": 1, "pageSize": 25, "pageCount": 1, "total": 1 } }}
Common cause of Strapi populate not working
If you get this error
{ "error": { "status": 403, "name": "ForbiddenError", "message": "Forbidden", "details": {} }}
It's Commonly caused by missing "find" permission in that collection or in the parents of that collection.
To fix it you can go to the Settings -> Users & Permission plugin -> Roles -> Public.
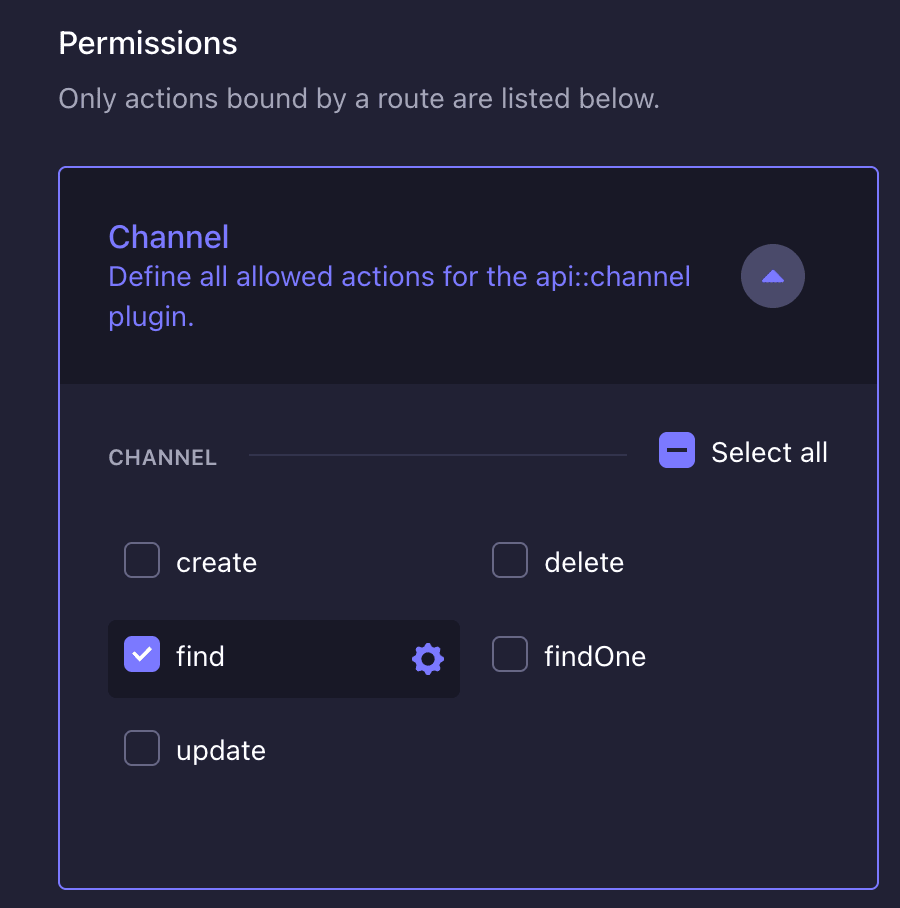